The Three Layers of a Web Application
All applications can be broken into three layers. Each layer has its own components and functionality. The following figure depicts the various layers that constitute a Web application.
As displayed in the preceding figure, an application has the following three layers:
- Presentation layer: Consists of the interface through which the users interact with the application.
- Business logic layer: Consists of the components of the application that control the flow of execution and communication between the presentation layer and the data layer.
- Data layer: Consists of components that expose the application data stored in databases to the business logic layer.
Architecture of a Web Application
The architecture of a Web application is the manner in which layers are distributed and the way in which they communicate with each other. Most applications are built by using all the three layers.
An application can have one of the following types of architectures:
- Single-tier architecture
- Two-tier architecture
- Three-tier architecture
- N-tier architecture
Single-tier Architecture
In an application based on single-tier architecture, all the three layers are integrated together and can be installed on a single computer. If the application needs to be accessed on multiple computers, a separate installation is required on each computer. For example, Adobe Photoshop that is used to create and edit graphics is a standalone application based on the single-tier architecture. The following figure displays the single-tier architecture.
Two-tier Architecture
In an application based on two-tier architecture, the three layers are distributed over two tiers, a client and a server. The presentation layer resides on each client computer, the business logic layer resides either on the client or on the server, and the data access layer resides on the server.
Depending on the business requirements, an organization can have the following types of two-tier application architecture:
Fat client and thin server: The architecture in which the business logic layer resides on the client is known as the fat client and thin server architecture. In this architecture, the client accepts user requests and processes these requests on its own. The client communicates with the server only when the data for communication or archival needs to be sent to the server.
Fat server and thin client: The architecture in which the business logic layer resides on the server is known as the fat server and thin client architecture. In this architecture, the client accepts requests from the users and forwards the same to the server. Further, the server processes these requests and provides responses.
The two-tier architecture can be used when multiple users need to access and manipulate common data storage. For example, an application needs to be developed to store an organization’s employee details in a database. In addition, the application should allow the retrieval of employee details from the database. In this case, the employee details need to be entered and retrieved by using multiple computers. Therefore, installing a database, along with the application on each computer, may lead to storage of duplicate records. In addition, a user cannot retrieve the employee details stored on some other computer. To avoid these problems, the application can be installed on each computer. However, the database is installed on a single computer, and the same can be accessed by the applications on multiple computers.
In the preceding example, the presentation and business logic layers are integrated and installed on each computer. The applications installed on the computers send requests to the server (database) for the storage and retrieval of data. On the other hand, the server accepts the requests and responds accordingly. In this setup, the fat client and thin server architecture is used. The following figure displays the fat client and thin server architecture.
In the preceding setup, the server only processes the data processing requests sent by the clients. Therefore, data retrieval is fast. As the business logic resides on the client, the other applications installed on the clients respond slowly. Further, if the business logic needs to be updated, the updates need to be installed on each client, which is a time-consuming process.
To overcome these limitations, the business logic layer can be placed on the server along with the data layer. In this way, only the presentation layer will be available on the clients. This, in turn, results in a faster response from the other applications installed on the clients. In addition, if the business logic needs to be updated, the updates need to be installed at only one location, which is on the server, rather than on each client. Such a setup is an example of the thin client and fat server architecture.
The following figure displays the thin client and fat server architecture.
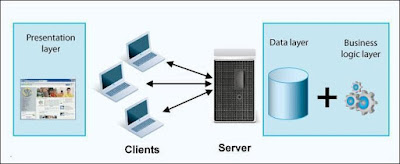
Three-tier Architecture
In an application based on three-tier architecture, the three layers of the application are placed separately as three different entities. This architecture is used for those applications in which merging the business logic layer with the presentation layer or the data layer may degrade the performance of the application. To improve the application performance, the three layers are kept separately and the communication among the layers occurs with the help of a request-response mechanism. The following figure displays the three-tier architecture
In the preceding figure, the business logic layer resides neither on a client nor on a database server. Instead, it resides on a separate server, which is known as an application server.
N-tier Architecture
The three-tier architecture is implemented when an effectively-distributed client/server architecture, which provides increased performance and scalability, is needed. However, it becomes a tedious task to design and deploy applications based on the three-tier architecture. The separation of presentation, business logic, and database access layers is not always obvious, because some business logic needs to appear on all the layers. Therefore, there is a need to further divide these layers. This requirement has led to the expansion of the three-tier application architecture to the N-tier application architecture.
A common approach to implement the N-tier architecture involves further separation of the presentation layer and the data layer. For example, the
presentation layer may be divided into the GUI layer and the presentation logic layer. Similarly, the data layer may be divided into the data access layer and the data layer.
The N-tier architecture separates the various business processes into discrete units of functionality called services. Each service implements a set of related business rules that defines what needs to be done and how it needs to be done within an organization.
The N-tier application architecture provides improved flexibility and scalability of applications, as compared to the three-tier application architecture. This is because the functioning of each layer is completely hidden from other layers, which makes it possible to change or update one layer without recompiling or modifying the other layers.